Random Number in Java: A Comprehensive Guide
Published on July 2nd, 2024
Creating random numbers is a fundamental task in programming, useful for various applications such as simulations, games, security, and more. In Java, generating random numbers can be achieved through several methods, each suitable for different use cases. This guide will take you from the basics of generating random numbers in Java to more advanced techniques, ensuring you have a thorough understanding of the topic. We’ll also touch on the significance of random number generation in various domains and how you can use the random number generator tool for practical applications.
What are Random Numbers
Random numbers are essential in many areas of computer science, from simulations and modeling to cryptography and gaming. A random number generator (RNG) is a system that produces a sequence of numbers that cannot be predicted better than by random chance. In Java, RNGs can be categorized into two main types: pseudo-random number generators (PRNGs) and secure random number generators (SRNGs).
- Pseudo-Random Number Generators (PRNGs): These use algorithms to produce sequences of numbers that only approximate true randomness. They are typically faster and suitable for most applications.
- Secure Random Number Generators (SRNGs): These are designed to be unpredictable and are used in security-critical applications like cryptography.
Basic Methods for Generating Random Numbers
Using Math.random()
The Math.random() method is the simplest way to generate random numbers in Java. This method returns a double value between 0.0 (inclusive) and 1.0 (exclusive). To use this method, simply call Math.random(), which will generate a random double value. While this method is easy to use, it has limitations, such as generating only double values and being less flexible compared to other methods.
Using java.util.Random
The java.util.Random class provides more flexibility and control over random number generation. It can generate different types of random numbers, such as int, float, long, and boolean. To use this class, instantiate an object of Random and then call the appropriate method, such as nextInt() for an integer or nextBoolean() for a boolean. This class is more versatile than Math.random() and is suitable for most general purposes.
Advanced Random Number Generation
Using java.security.SecureRandom
For cryptographic purposes, use java.security.SecureRandom, which provides a cryptographically strong random number generator. This class is part of the java.security package and is designed to produce random numbers that are secure and unpredictable, making it ideal for tasks like generating keys for encryption. To use SecureRandom, create an instance of the class and call methods similar to those in Random, but with the added assurance of security.
Using java.util.concurrent.ThreadLocalRandom
For concurrent applications, java.util.concurrent.ThreadLocalRandom is an excellent choice. This class is part of the java.util.concurrent package and is designed for use in multi-threaded environments. It reduces contention by providing each thread with its own random number generator. To use ThreadLocalRandom, call its static methods directly without needing to instantiate an object, which simplifies its usage in concurrent programming.
Generating Random Numbers within a Range
To generate random numbers within a specific range, such as between a minimum and maximum value, you can use a combination of basic and advanced methods. For example, by using java.util.Random or Math.random(), you can scale and shift the random values to fit the desired range. This involves multiplying the random value by the range width and then adding the minimum value. The Random class's nextInt(int bound) method directly supports generating integers within a specific range by specifying the upper bound.
Generating Random Numbers for Specific Data Types
Different applications may require random numbers of various data types. The java.util.Random class supports generating random integers, doubles, floats, longs, and booleans through its various methods. Each method provides a random value of the corresponding type. For instance, nextInt() generates a random integer, while nextDouble() generates a random double. By choosing the appropriate method, you can easily generate random values of the needed type.
Seeding Random Number
Seeding is crucial for reproducibility in random number generation. By providing the same seed value to a random number generator, you can produce the same sequence of random numbers, which is helpful for debugging and testing. In Java, both java.util.Random and java.security.SecureRandom allow you to specify a seed value when instantiating the object. This seed initializes the generator's internal state, ensuring that the sequence of generated numbers can be replicated if the same seed is used again.
Performance Considerations
The performance of random number generation can be a critical factor, especially in applications requiring a high volume of random numbers, such as simulations or games. Math.random() and java.util.Random are generally fast and suitable for most purposes. However, in multi-threaded applications, ThreadLocalRandom is preferred due to its reduced contention. On the other hand, SecureRandom is slower but necessary for security-critical applications where unpredictability is paramount.
Practical Applications of Random Number Generation
Random number generation has a wide range of practical applications, including:
- Simulations: Random numbers are used to model complex systems and predict outcomes in simulations.
- Gaming: Randomness enhances game unpredictability and excitement by randomizing elements like loot drops and enemy behavior.
- Cryptography: Secure random numbers are essential for generating encryption keys, initialization vectors, and other cryptographic parameters.
- Statistical Sampling: Random sampling is used in statistics to draw unbiased samples from a population for analysis.
- Machine Learning: Random initialization of weights and data shuffling are common practices in training machine learning models.
Using tools like the random number generator tool can help streamline these applications, providing quick and easy access to random number generation for various needs.
Common Pitfalls and Best Practices
When working with random numbers in Java, it is essential to be aware of common pitfalls and follow best practices to ensure reliable and predictable behavior:
- Avoid Reusing Random Instances: Creating multiple instances of Random without proper seeding can lead to duplicate sequences. Use a single instance or consider ThreadLocalRandom for multi-threaded environments.
- Use SecureRandom for Security: For cryptographic purposes, always use SecureRandom instead of Random to ensure the randomness is strong enough for security applications.
- Consider Performance Implications: Choose the appropriate random number generator based on the performance needs of your application. Use Math.random() or Random for general purposes, and ThreadLocalRandom for concurrent applications.
Conclusion
Generating random numbers in Java is a versatile and powerful capability that supports a wide array of applications. From simple random number generation using Math.random() and java.util.Random to more advanced techniques with SecureRandom and ThreadLocalRandom, Java provides robust tools for various needs. Understanding how to generate random numbers within a range, for specific data types, and with proper seeding allows you to harness the full potential of randomness in your programs. By following best practices and being aware of common pitfalls, you can ensure that your random number generation is both efficient and reliable. Utilize tools like the random number generator tool to further enhance your applications with random numbers.
Frequently Asked Questions (FAQs)
- What is a random number generator (RNG) in Java?
A random number generator in Java is a tool or algorithm that produces a sequence of numbers that cannot be predicted logically. Java provides several classes for random number generation, such as Math.random(), java.util.Random, java.security.SecureRandom, and java.util.concurrent.ThreadLocalRandom.
- How does Math.random() work in Java?
Math.random() generates a double value between 0.0 (inclusive) and 1.0 (exclusive). This method provides a quick way to generate a random number but only returns double values.
- What is java.util.Random?
java.util.Random is a class in Java that provides methods to generate random numbers of various types, including int, float, long, and boolean.
- When should I use java.security.SecureRandom?
Use java.security.SecureRandom for cryptographic applications where the randomness needs to be unpredictable and secure, such as generating encryption keys or tokens.
- What is java.util.concurrent.ThreadLocalRandom used for?
java.util.concurrent.ThreadLocalRandom is designed for use in multi-threaded environments. It reduces contention by providing each thread with its own instance of the random number generator.
- How can I generate a random integer in Java?
You can generate a random integer using the nextInt() method of the java.util.Random class.
- Can I generate random numbers within a specific range in Java?
Yes, by using methods such as nextInt(int bound) in java.util.Random or scaling and shifting the output of Math.random(), you can generate random numbers within a specific range.
- How do I generate a random boolean in Java?
Use the nextBoolean() method of the java.util.Random class to generate a random boolean value.
- What is seeding in random number generation?
Seeding initializes the random number generator's internal state. Providing the same seed value will produce the same sequence of random numbers, which is useful for debugging and testing.
- Why is seeding important?
Seeding ensures reproducibility, meaning that you can generate the same sequence of random numbers if the same seed is used again.
- How do I seed a java.util.Random instance?
Pass a long value as a seed to the Random constructor, e.g., new Random(12345L).
- Is Math.random() suitable for all applications?
No, Math.random() is suitable for simple applications but lacks the flexibility and security required for more complex or cryptographic tasks.
- How can I generate a random double in Java?
Use the nextDouble() method of the java.util.Random class to generate a random double value.
- What are pseudo-random numbers?
Pseudo-random numbers are numbers generated by an algorithm that simulates randomness. They are not truly random but are sufficient for most practical purposes.
- What is the difference between Random and SecureRandom?
Random provides fast, pseudo-random number generation for general purposes, while SecureRandom offers cryptographically strong random numbers suitable for security-sensitive applications.
- Can I generate random floating-point numbers in Java?
Yes, you can use methods like nextFloat() and nextDouble() in the java.util.Random class to generate random float and double values, respectively.
- How do I generate random bytes in Java?
Use the nextBytes(byte[] bytes) method of the java.util.Random class to fill a byte array with random bytes.
- What is ThreadLocalRandom best used for?
ThreadLocalRandom is best used in multi-threaded environments to reduce contention and improve performance by providing thread-local random number generation.
- How do I generate a random long value in Java?
Use the nextLong() method of the java.util.Random class to generate a random long value.
- What are the limitations of Math.random()?
Math.random() only generates double values and does not offer methods for generating other types of random numbers or for specifying ranges directly.
- How can I generate a random number within a specific range using Math.random()?
Multiply the result of Math.random() by the range width and add the minimum value of the range to scale and shift the random number into the desired range.
- Is java.util.Random thread-safe?
No, java.util.Random is not thread-safe. For multi-threaded applications, use java.util.concurrent.ThreadLocalRandom.
- How do I generate a random integer within a specific range?
Use the nextInt(int bound) method of the java.util.Random class, which generates a random integer between 0 (inclusive) and the specified bound (exclusive).
- What is a secure random number generator (SRNG)?
An SRNG, like java.security.SecureRandom, produces random numbers that are cryptographically secure, meaning they are unpredictable and suitable for security applications.
- Can I control the randomness of SecureRandom?
Yes, you can seed a SecureRandom instance to control its randomness, similar to how you would seed a Random instance.
- How do I generate random alphanumeric strings in Java?
Combine random number generation with character arrays containing alphanumeric characters. Select random indices from the array to construct the string.
- What are the performance considerations for random number generation?
Consider the speed and thread-safety of the random number generator. Math.random() and Random are generally fast, while SecureRandom is slower but secure.
- How can I ensure my random numbers are truly random?
For most applications, pseudo-random numbers are sufficient. For cryptographic or security-sensitive tasks, use SecureRandom.
- What is a seed value in random number generation?
A seed value is an initial value used to start the random number generation process, ensuring reproducibility of the random sequence.
- How do I generate a random index for an array in Java?
Use the nextInt(int bound) method of the Random class, where the bound is the length of the array.
- Can I generate random numbers in parallel in Java?
Yes, using java.util.concurrent.ThreadLocalRandom allows for efficient random number generation in multi-threaded environments.
- Why should I use SecureRandom for cryptography?
SecureRandom ensures that the random numbers are unpredictable, a crucial requirement for cryptographic applications.
- How do I generate a random UUID in Java?
Use the java.util.UUID class's randomUUID() method to generate a universally unique identifier (UUID).
- What is the difference between nextInt() and nextInt(int bound) in Random?
nextInt() generates a random integer within the full range of int values, while nextInt(int bound) generates a random integer between 0 (inclusive) and the specified bound (exclusive).
- How do I generate random decimal numbers in Java?
Use the nextDouble() method in the Random class for generating random decimal (double) numbers.
- Can SecureRandom be used for non-cryptographic purposes?
Yes, but it is generally slower than Random due to its additional security features. Use SecureRandom only when security is a concern.
- How can I generate a random number for a lottery simulation?
Use java.util.Random to generate random integers within the range of lottery numbers, ensuring each number is unique.
- Is ThreadLocalRandom faster than Random in multi-threaded environments?
Yes, ThreadLocalRandom reduces contention and is faster in multi-threaded environments compared to Random.
- How do I reset a Random instance?
Create a new Random instance with the desired seed value to reset the sequence of random numbers.
- What is the purpose of the nextGaussian() method in Random?
The nextGaussian() method generates random numbers with a Gaussian (normal) distribution, centered at 0 with a standard deviation of 1.
- How can I generate random numbers in a specific format, like currency?
Generate the random number and then format it as needed using Java's formatting classes, such as DecimalFormat.
- How do I avoid generating the same random number twice?
Track previously generated numbers and re-generate if a duplicate is found. For small ranges, consider using a list of numbers and shuffling it.
- What are the benefits of using ThreadLocalRandom?
ThreadLocalRandom reduces contention in multi-threaded applications and improves performance by providing thread-local instances of the random number generator.
- Can Random be used for generating secure passwords?
No, use SecureRandom for generating secure passwords to ensure unpredictability and security.
- How do I generate random dates in Java?
Generate random long values within the range of timestamps and convert them to Date objects.
Hire the best without stress
Ask us how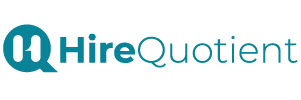
Never Miss The Updates
We cover all recruitment, talent analytics, L&D, DEI, pre-employment, candidate screening, and hiring tools. Join our force & subscribe now!
Stay On Top Of Everything In HR